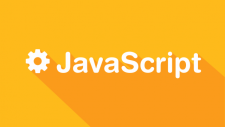
Recently I was faced with a situation where I needed to get the value of an HTML5 date input field when it was changed by the user. My first reaction was to setup a JavaScript listener that listens for a change or a click on the input element and then grab the value of the date accordingly. So I setup my listener with jQuery and with vanilla JavaScript but I was unsuccessful in getting consistent result values no matter how I setup the listener. Finally, as a solution, I stumbled upon the blur() method to register an event to fire when the input field looses focus. From here I was able to gather value of the source element and then get the value from there. Below is an example of the code I am using to do this. Please, if you have a suggestion or a question, I am all ears and would love to hear from you.
<!-- The date input field --> <input type="date" id="myDateField" /> <script type="text/javascript"> // The function get the date value when the date input looses focus function getMyDateValue(e) { // Get the date value from the srcElement of the event var dateArr = e.srcElement.value.split('-'); // Make sure we are dealing with an array of at least length 2 if (dateArr.length > 1) { // Format the date as needed // Currently mm/dd/yyyy console.log(dateArr[1] + '/' + dateArr[2] + '/' + dateArr[0]); } } // Add an event listener to my date field document.getElementById("myDateField").addEventListener("blur", getMyDateValue) </script>